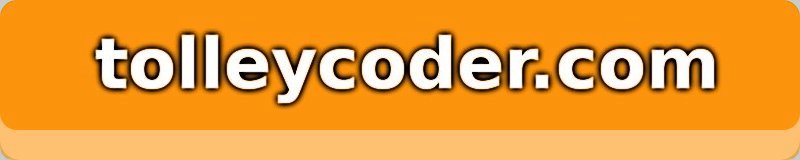
Download the actual object here, read on for instructions.
One of the problems with AJAX is that it breaks your back button. If you are on a page and you make several changes by clicking things and expanding things and then hit the back button, all of these changes will normally be lost. If you try to hit the forward button you will receive the page in its original state. On the right kind of page, this really isn't a problem, but on most pages people expect a certain kind of behaviour. They expect to be able to hit the back and forth buttons and when it doesn't work they feel like your site is "broken".
In order to combat this, I've written a simple History object, which can be downloaded here. Its a simple javascript object that uses the location.hash property of the document to store changes caused by the user. As you browse thru my projects section you will see it in action. Pay attention to the end of the URL in the address bar. When you click on a project, "#projectid=X" should appear at the end of the URL and the browser will put the new information on its history stack.
Before I get into my history object I'd like to point out a few things. This object doesn't store the order of events. All it does is store variables and their values at the end of the URL. If you use this object, don't expect it to magically recreate your page state for you when you go back and forth thru the browser histroy. You will have to write code to do something with the information you store in the history.
With that being said, its time to show you how to use it. The way I ususally use it is to write a function and plug it into the window's onload event, like so:
<script language="javascript">
window.onload = function()
{
// Create and initialize the history object
window.History = new History;
window.History.initialize();
}// End window.onload
</script>
I set window.History equal to a new History object so all my other javascript will have access to it. When the new history object is initialized it will check the location.hash variable, which is built into you browser. If there is anything stored there, the History object will break it up like a query string and store the values in an associative array for quick and easy access later.
When your page is first loaded there shouldn't be anything in the hash. You have to add something to it based on user actions. One example you can see in action right now is when a user clicks on a project label on the left it calls a function to send a request to my server to get the project. I also call window.History.add( 'projectid', X ), where X identifies the project that the user wants to view.
If you want to remove something from the history, you can call window.History.remove( 'blah' ) where 'blah' is the name of the field you want to remove. This will remove it from the history and reset location.hash to reflect your change.
So, we have all the fields and their values that we want to keep track of, what now? Well, the way I do it is in the window.onload function we talked about I'll do something like
var qryStr = window.History.getQryStr();
getQryStr returns any information that is stored in the History and returns it formatted like a query string. I can then test for the length of that string, and if its greater than zero, I will send it to the server to restore the page state:
if( qryStr.length > 0 ) {
// Do an ajax request using
// qryStr as the post fields.
}// End if
Of course, you will have to have something on the server to expect these variables, and then you will have to write yet more javascript to process the return of the call, but thats the fun part. Theres two other functions in the History object, but I'll let you the viewer figure them out. They are well commented and pretty small function.